I want to share CODE for Arduino uno with Ethernet shield web page for temperature monitor.
( 3 DS18B20 sensors and 1 DHT22 )
In PRTG i use HTTP Content Sensor with 5 number of channel.
Code :
#include <DHT.h>
#define DHTPIN 3 // connecting DHT sensor pin to pin 2 on our Arduino
#define DHTTYPE DHT22 // we are using DHT sensor 22
DHT dht(DHTPIN, DHTTYPE);
#include <SPI.h>
#include <Ethernet.h>
#include <LiquidCrystal.h>
#include <OneWire.h>
#include <DallasTemperature.h>
#include <Wire.h>
#define ONE_WIRE_BUS 2 //definiramo pin, na katerega so priklučeni senzorju DS18B20
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
DeviceAddress Thermometer1 = { 0x28, 0x80, 0xD3, 0x1E, 0x00, 0x00, 0x80, 0x45 };//ok
DeviceAddress Thermometer2 = { 0x28, 0xFF, 0x47, 0xA3, 0x71, 0x16, 0x05, 0xEC };//ok
DeviceAddress Thermometer3 = { 0x28, 0xB1, 0xEE, 0x1E, 0x00, 0x00, 0x080, 0xF7 };//ok
LiquidCrystal lcd(3, 4, 5, 6, 7, 8); //the pins used for the lcd display
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xEC }; //mac address of ethernet shield
IPAddress ip(192,168,5,90); // ip address of ethernet shield
EthernetServer server(80); //sets the port for the ethernet shield- eg: port 80 http
void setup() {
Serial.begin(9600);
lcd.begin(16, 2); // change this back to 16, 2
dht.begin();
Ethernet.begin(mac, ip);
server.begin();
Serial.print("Server address:"); //prints server address to serial connection
Serial.println(Ethernet.localIP());
}
void loop() {
delay(2000);
float h = dht.readHumidity();
float t = dht.readTemperature();
float temp1 = sensors.getTempC(Thermometer1);
float temp2 = sensors.getTempC(Thermometer1);
float temp3 = sensors.getTempC(Thermometer1);
if (isnan(t) || isnan(h)) {
Serial.println("Count not detect sensor");
} else { //prints sensor output to lcd and serial monitor
lcd.setCursor(0, 0);
lcd.print("Temp: ");
lcd.setCursor(10, 0);
lcd.print(t);
lcd.setCursor(0, 1);
lcd.print("Humidity: ");
lcd.setCursor(10, 1);
lcd.print(h);
Serial.print('\n');
Serial.print("Senzor DHT 22 - PIN 3");
Serial.print('\n');
Serial.print("Vlaznost: ");
Serial.print(h);
Serial.print(" %\t");
Serial.print('\n');
Serial.print("Temperatura: ");
Serial.print(t);
Serial.println(" *C");
Serial.print('\n');
Serial.print("Senzorji DS18B20 - PIN 2 ");
Serial.print('\n');
Serial.print("Temperature 1: ");
Serial.println(temp1);
Serial.print("Temperature 2: ");
Serial.println(temp2);
Serial.print("Temperature 3: ");
Serial.println(temp3);
Serial.print("--------------------------------- ");
Serial.print("ponovno berem temperaturo..... ");
Serial.print('\n');
}
EthernetClient client = server.available();
if (client) {
Serial.println("new client");
boolean blankLine = true;
while (client.connected()) {
if (client.available()) {
char c = client.read();
Serial.write(c);
if (c == '\n' && blankLine) { //initializing HTTP
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close"); // the connection will be closed after completion of the response
client.println("Refresh: 5"); // refresh the page automatically every 5 sec
client.println();
client.println("<!DOCTYPE HTML>");
client.println("<html>");
client.println("<center>");
client.println("<H1>");
client.println("Current Readings:");
client.println("</H1>");
client.println("<H2>");
client.println("Temperature: ");
client.println("</H2>");
client.println("<H1>");
client.print("[");
client.print(t);
client.println("] °");
client.println("C");
client.println("</H1>");
client.println("<br />");
client.println("<H2>");
client.println("Humidity: ");
client.println("</H2>");
client.println("<p />");
client.println("<H1>");
client.print("[");
client.print(h);
client.print("] %\t");
client.println("</H1>");
client.println("<p />");
client.println("</center>");
client.println("</html>");
sensors.requestTemperatures(); //Get temperature of all sensors
client.print ("<font color=black size=7>");
client.println("<br />");
client.print ("<font color=black size=7>");
client.print("Senzor 1: ");
client.print("[");
client.print(sensors.getTempC(Thermometer1)); //print temperature from DS18x20 sensor
client.println("] °");
client.println("C");
client.print ("<font color=green size=7>");
client.print ("<font color=black size=7>");
client.println("<br />");
client.print ("<font color=black size=7>");
client.print("Senzor 2: ");
client.print("[");
client.print(sensors.getTempC(Thermometer2)); //print temperature from DS18x20 sensor
client.println("] °");
client.println("C");
client.print ("<font color=green size=7>");
client.print ("<font color=black size=7>");
client.println("<br />");
client.print ("<font color=black size=7>");
client.print("Senzor 3: ");
client.print("[");
client.print(sensors.getTempC(Thermometer3)); //print temperature from DS18x20 sensor
client.println("] °");
client.println("C");
client.print ("<font color=green size=7>");
break;
}
if (c == '\n') {
blankLine = true;
}
else if (c != '\r') {
blankLine = false;
}
}
}
delay(1);
client.stop();
Serial.println("Disonnected");
}
}
Regards , Damjan
Screenshots
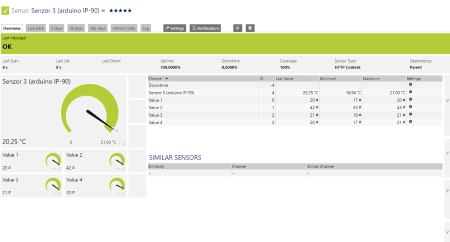
Click here to enlarge.
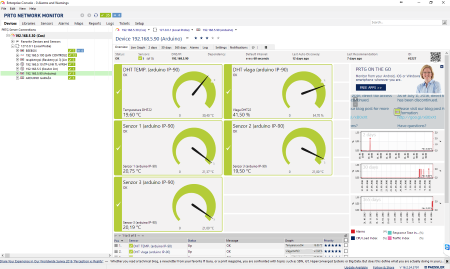
Click here to enlarge.
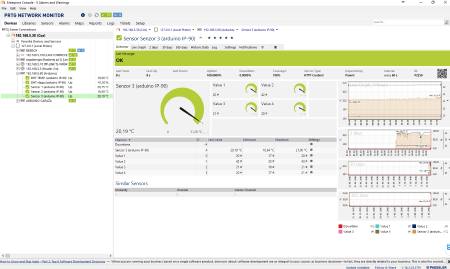
Click here to enlarge.
Add comment